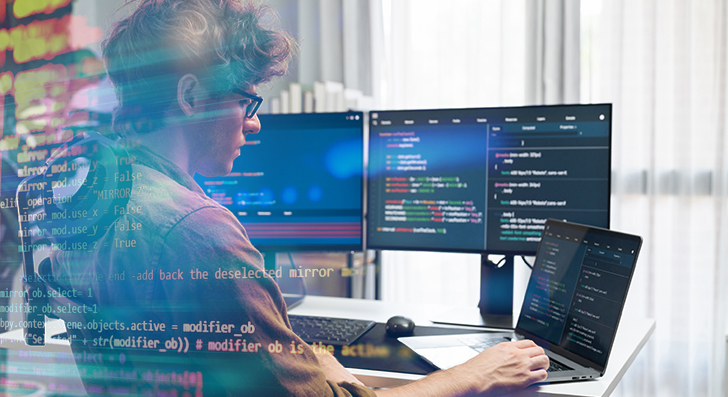
Scalability usually means your application can handle advancement—additional end users, a lot more data, and more targeted visitors—devoid of breaking. Being a developer, developing with scalability in your mind saves time and stress afterwards. In this article’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not a little something you bolt on afterwards—it should be section of your respective strategy from the start. Numerous purposes fail if they improve quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your program will behave under pressure.
Start out by creating your architecture being flexible. Keep away from monolithic codebases where by every little thing is tightly linked. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent areas. Each individual module or services can scale on its own with out impacting The full procedure.
Also, consider your database from day just one. Will it need to handle 1,000,000 users or perhaps 100? Select the suitable type—relational or NoSQL—according to how your info will improve. Approach for sharding, indexing, and backups early, even if you don’t need to have them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work less than present-day disorders. Take into consideration what would come about If the consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like concept queues or occasion-driven methods. These assist your app handle more requests with out receiving overloaded.
Once you build with scalability in your mind, you are not just planning for achievement—you might be lowering future complications. A nicely-prepared program is simpler to keep up, adapt, and improve. It’s better to prepare early than to rebuild later on.
Use the appropriate Databases
Choosing the ideal databases is often a essential Section of making scalable purposes. Not all databases are built the identical, and utilizing the Mistaken one can gradual you down as well as bring about failures as your app grows.
Start by comprehension your data. Could it be hugely structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. They're robust with relationships, transactions, and regularity. Additionally they assistance scaling procedures like read through replicas, indexing, and partitioning to manage a lot more site visitors and information.
In the event your facts is more adaptable—like consumer exercise logs, merchandise catalogs, or documents—consider a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at dealing with big volumes of unstructured or semi-structured information and can scale horizontally far more conveniently.
Also, take into consideration your browse and create patterns. Will you be doing lots of reads with much less writes? Use caching and read replicas. Are you currently managing a large write load? Take a look at databases which will handle large write throughput, or perhaps event-based mostly details storage devices like Apache Kafka (for short term details streams).
It’s also wise to think forward. You may not have to have Superior scaling features now, but selecting a database that supports them indicates you gained’t require to change afterwards.
Use indexing to speed up queries. Keep away from avoidable joins. Normalize or denormalize your facts based on your accessibility patterns. And usually check database performance as you develop.
In a nutshell, the ideal databases depends on your application’s construction, pace needs, And the way you be expecting it to grow. Take time to pick sensibly—it’ll help save plenty of problems afterwards.
Enhance Code and Queries
Speedy code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Start off by creating clean, very simple code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t choose the most complex Option if an easy 1 is effective. Maintain your functions shorter, centered, and simple to test. Use profiling instruments to seek out bottlenecks—locations where by your code normally takes as well extensive to run or uses an excessive amount memory.
Up coming, look at your databases queries. These typically slow factors down greater than the code alone. Make certain each question only asks for the data you really have to have. Keep away from SELECT *, which fetches all the things, and alternatively find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
When you discover the exact same data remaining requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached and that means you don’t really have to repeat expensive operations.
Also, batch your database functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app far more successful.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred records may crash if they have to take care of one million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and much more visitors. If every little thing goes by means of one particular server, it is going to speedily become a bottleneck. That’s where by load balancing and caching are available. Both of these instruments enable keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing many of the function, the load balancer routes users to different servers dependant on availability. This suggests no solitary server will get overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to build.
Caching is about storing knowledge briefly so it may be reused quickly. When buyers ask for exactly the same information all over again—like an item web page or simply a profile—you don’t should fetch it from your databases whenever. You'll be able to serve it within the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) shops static data files close to the person.
Caching lowers database load, enhances velocity, and helps make your application much more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are uncomplicated but potent instruments. Together, they help your application handle a lot more buyers, stay rapidly, and Get better from issues. If you intend to mature, you'll need both equally.
Use Cloud and Container Applications
To develop scalable purposes, you'll need equipment that permit your application develop simply. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and providers as you require them. You don’t really have to buy hardware or guess long term capacity. When site visitors will increase, it is possible to insert extra means with just some clicks or automatically using auto-scaling. When traffic drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. It is possible to target constructing your app as opposed to handling infrastructure.
Containers are An additional important tool. A container offers your application and almost everything it has to operate—code, libraries, options—into a single unit. This can make it uncomplicated to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to control them. Kubernetes handles deployment, scaling, and recovery. If just one portion of one's app crashes, it restarts it mechanically.
Containers also ensure it is simple to independent elements of your application into providers. You are able to update or scale parts independently, which happens to be perfect for overall performance and trustworthiness.
In a nutshell, utilizing cloud and container resources usually means you'll be able to scale fast, deploy quickly, and Recuperate immediately when difficulties materialize. If you need your application to increase without boundaries, get started making use of these applications early. They preserve time, cut down threat, and assist you remain focused on creating, not correcting.
Monitor Almost everything
If you don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, spot concerns early, and make superior conclusions as your app grows. It’s a crucial Component of building scalable programs.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—check your app way too. Control how much time it's going to take for buyers to load pages, how often errors take place, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s going on within your code.
Build alerts for vital problems. For instance, In case your response time goes above a limit or perhaps a services goes down, you need to get notified instantly. This assists you repair problems quick, frequently prior to customers even observe.
Monitoring can also be useful after you make variations. When you deploy a whole new characteristic and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic hurt.
As your app grows, targeted visitors and facts enhance. Without having checking, you’ll miss out on signs of hassle until eventually it’s also late. But with the correct tools set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about being familiar with your program and ensuring that it works well, even under pressure.
Remaining Ideas
Scalability isn’t only click here for huge providers. Even tiny applications want a solid foundation. By planning cautiously, optimizing sensibly, and using the suitable resources, you may Develop applications that grow easily without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.